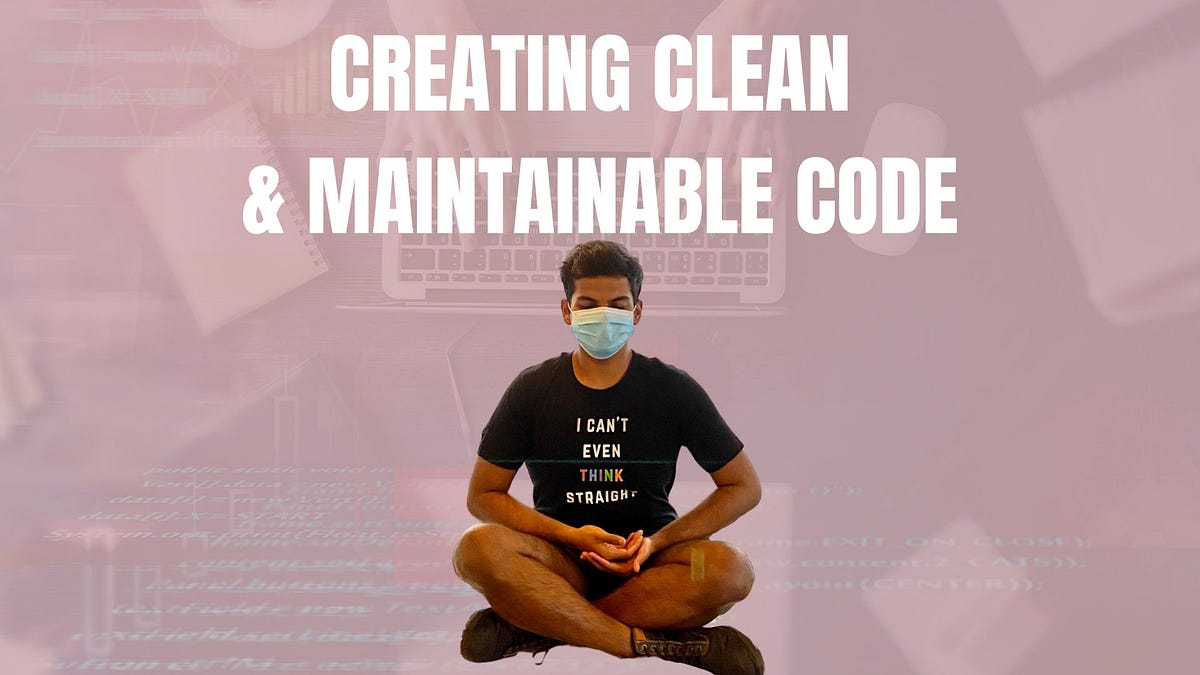
Writing Clean And Maintainable Code: A Developer’s Handbook
In the dynamic realm of software development, the ability to write clean and maintainable code is akin to wielding a masterful brush in the realm of art. Clean code not only makes your software more readable but also streamlines collaboration, eases maintenance, and enhances the overall development experience. Let’s embark on a journey to uncover the principles and practices that constitute the art of writing clean and maintainable code.
1. Meaningful Naming:
- Descriptive and Pronounceable: Choose names for variables, functions, and classes that convey their purpose clearly and are easy to pronounce.
- Avoid Ambiguity: Eliminate ambiguity by avoiding single-letter names or abbreviations unless they are widely recognized.
python
# Unclear
def f(x):
s = 0
for i in x:
s += i
return s
# Clear
def calculate_sum(numbers):
total_sum = 0
for number in numbers:
total_sum += number
return total_sum
2. Consistent Formatting:
- Follow a Style Guide: Adhere to a consistent coding style guide, whether it’s PEP 8 for Python, Google Style Guide for Java, or another relevant standard for your chosen language.
- Indentation and Spacing: Maintain consistent indentation and spacing for improved readability.
java
// Inconsistent Formatting
public class Example {
public void displayInfo() {
System.out.println("Hello, world!");
}
}
// Consistent Formatting
public class Example {
public void displayInfo() {
System.out.println("Hello, world!");
}
}
3. SOLID Principles:
- Single Responsibility Principle (SRP): Each class or module should have only one reason to change.
- Open/Closed Principle (OCP): Entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes should be substitutable for their base types.
- Interface Segregation Principle (ISP): A class should not be forced to implement interfaces it does not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules but both should depend on abstractions.
4. Comments for Why, Not What:
- Express Intent: Use comments to explain why code is written, focusing on the rationale behind decisions, rather than explaining what the code does.
- Avoid Redundancy: Write self-explanatory code and use comments sparingly to provide insights that are not evident from the code itself.
javascript
// Bad Comment: Unnecessary and redundant
function add(a, b) {
// Add a and b
return a + b;
}
// Good Comment: Provides context and rationale
function calculateTotalPrice(price, quantity) {
// Multiply the price by the quantity to get the total price
return price * quantity;
}
5. Modularization and Functions:
- Single Responsibility Principle (Function Level): Functions should have a single responsibility, making them concise and focused.
- Avoid Global State: Minimize the use of global variables and aim for encapsulation within functions and classes.
python
# Non-modular and complex function
def process_data(data):
# Perform data processing, validation, and manipulation
result = ...
# Save data to the database
save_to_database(result)
# Notify users
notify_users(result)
# Modular and focused functions
def process_data(data):
processed_data = validate_and_manipulate(data)
return processed_data
def save_to_database(data):
# Save data to the database
def notify_users(data):
# Notify users
6. Test-Driven Development (TDD):
- Write Tests First: Begin by writing tests before implementing functionality, ensuring that code meets specified requirements.
- Continuous Refactoring: Refactor code continuously while keeping tests passing, allowing for incremental improvements without compromising functionality.
7. Continuous Refactoring:
- Refactor Ruthlessly: Regularly revisit and refactor code to improve its structure, readability, and adherence to best practices.
- Code Reviews: Engage in code reviews with peers to identify areas for improvement and collectively enhance code quality.
Conclusion:
Writing clean and maintainable code is not just a technical endeavor but an art form that combines creativity, discipline, and collaboration. By following these principles and practices, developers can craft code that stands the test of time, fostering a positive development culture and contributing to the success of software projects.
As you embark on your coding journey, remember that the true beauty of code lies not just in its functionality but in its elegance, simplicity, and ease of comprehension.