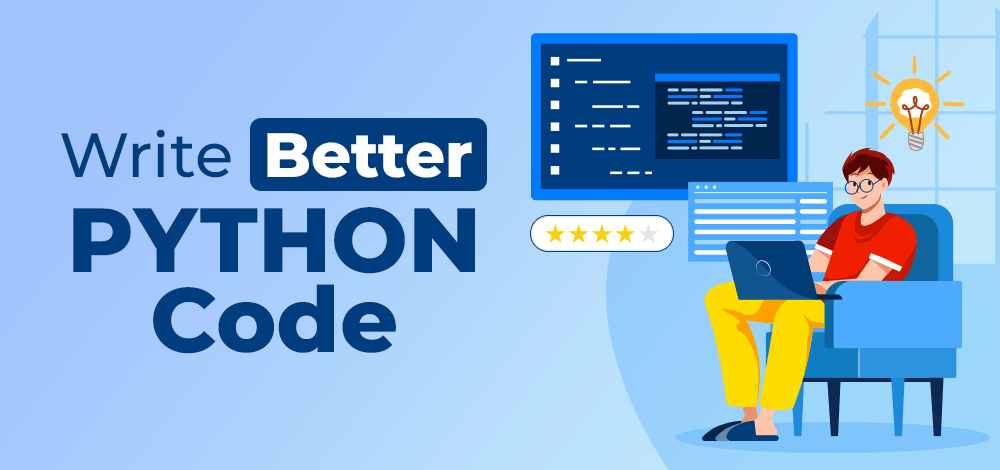
Python Tricks for Efficient Data Manipulation: Code Examples Included
Python is a versatile programming language known for its readability and ease of use. When it comes to data manipulation, Python provides a powerful set of tools and libraries. In this guide, we’ll explore some tricks and techniques for efficient data manipulation in Python, with code examples included.
1. List Comprehensions
List comprehensions are a concise and expressive way to create lists. They can also be used for simple data manipulation tasks. For instance, to create a list of squares:
python
squares = [x2 for x in range(10)]
print(squares)
2. Lambda Functions
Lambda functions are anonymous, one-line functions. They are handy for short, simple operations, especially when used with functions like `map` or `filter`. Example:
python
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x2, numbers))
print(squared)
3. Zip and Unpack
The `zip` function combines two or more iterables element-wise. You can also use the “ operator to unpack elements. For example:
python
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
combined = list(zip(names, ages))
print(combined)
4. Dictionary Comprehensions
Similar to list comprehensions, you can use dictionary comprehensions to create dictionaries in a concise way. Example:
python
fruits = ['apple', 'banana', 'cherry']
fruit_lengths = {fruit: len(fruit) for fruit in fruits}
print(fruit_lengths)
5. Enumerate
The `enumerate` function adds a counter to an iterable. It returns tuples containing the index and the item. Example:
python
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
6. Collections.Counter
The `Counter` class from the `collections` module is excellent for counting occurrences of elements in a list. Example:
python
from collections import Counter
colors = ['red', 'blue', 'red', 'green', 'blue', 'red']
color_counts = Counter(colors)
print(color_counts)
7. Unpacking with Asterisks
Asterisks can be used for unpacking elements from iterables, especially in function arguments. Example:
python
def multiply(numbers):
result = 1
for num in numbers:
result = num
return result
print(multiply(2, 3, 4)) Output: 24
8. Pandas for DataFrames
When working with tabular data, the `pandas` library is a go-to tool. It provides a `DataFrame` for efficient data manipulation. Example:
python
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Conclusion
These Python tricks and techniques offer a glimpse into the efficient world of data manipulation. Whether you’re dealing with lists, dictionaries, or more complex tabular data, Python provides a variety of tools to streamline your coding process. Experiment with these examples and incorporate them into your data manipulation tasks for improved efficiency. Happy coding!