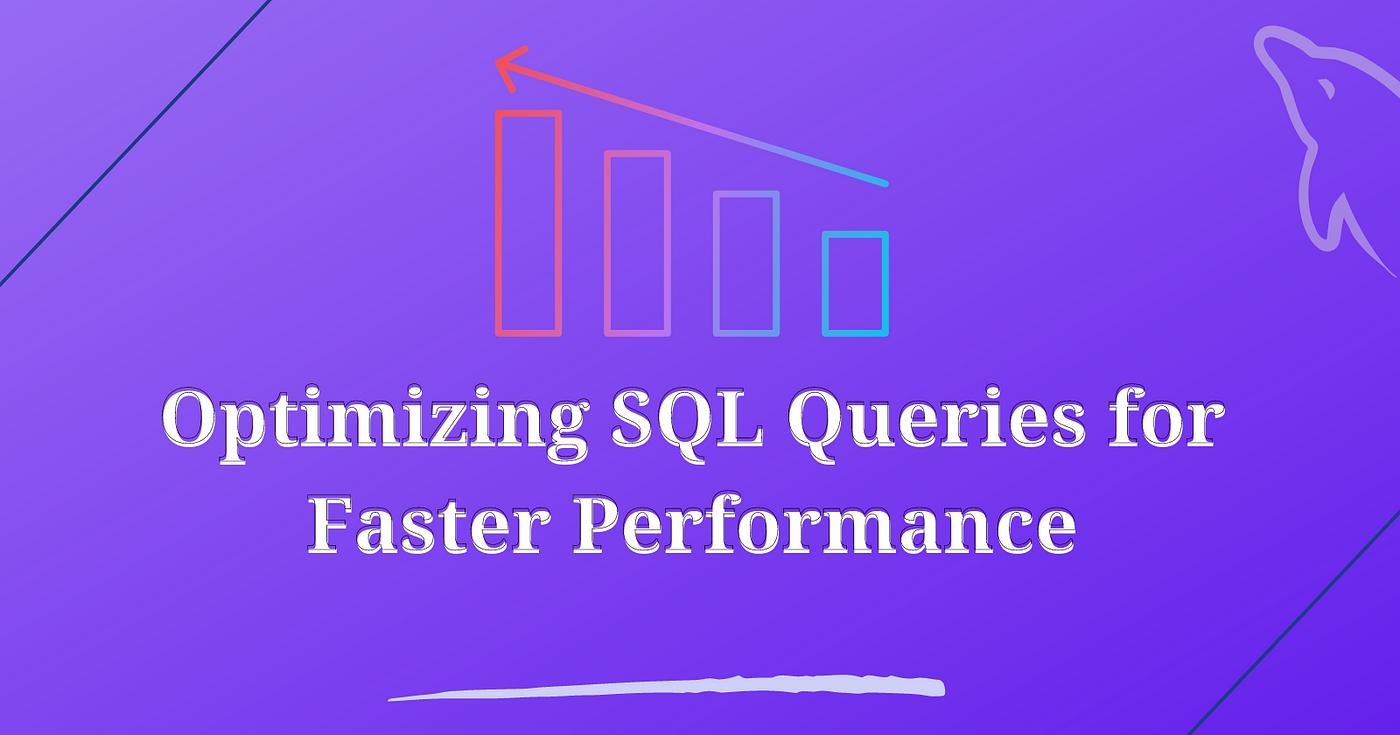
Optimizing SQL Queries: A Developer’s Guide with Real Code Samples
In the realm of database-driven applications, the efficiency of SQL queries is paramount to ensuring optimal performance. In this guide, we’ll explore strategies for optimizing SQL queries with real code samples. Whether you’re dealing with large datasets or complex queries, these techniques will help you fine-tune your SQL skills and enhance the speed of your database operations.
Why Optimize SQL Queries?
Optimizing SQL queries is crucial for several reasons:
1. Faster Response Times: Well-optimized queries result in quicker responses, leading to a more responsive and efficient application.
2. Reduced Server Load: Efficient queries consume fewer server resources, leading to lower hosting costs and better scalability.
3. Improved User Experience: Faster queries translate to smoother user experiences, contributing to higher user satisfaction.
4. Scalability: Optimized queries are essential for applications that need to scale with growing data volumes.
Identifying Performance Bottlenecks
Before diving into optimization techniques, it’s essential to identify potential bottlenecks. Common signs of inefficient queries include:
– Slow Query Execution: Queries taking longer than expected to execute.
– Excessive Resource Consumption: High CPU or memory usage during query execution.
– Long Query Execution Plans: Complex and convoluted execution plans.
Optimization Techniques with Real Code Samples
1. Use Indexes Wisely:
– Issue: Lack of proper indexing can significantly slow down query performance.
– Solution:
– Identify columns used in WHERE clauses and JOIN conditions.
– Create indexes on these columns.
sql
-- Creating an index on a column
CREATE INDEX idx_username ON users(username);
2. Optimize JOIN Operations:
– Issue: Inefficient JOINs can lead to performance degradation.
– Solution:
– Use INNER JOIN when only matching rows are needed.
– Avoid SELECT ; explicitly list columns to retrieve.
sql
-- Using INNER JOIN
SELECT orders.order_id, customers.customer_name
FROM orders
INNER JOIN customers ON orders.customer_id = customers.customer_id;
3. Filter Data with WHERE Clause:
– Issue: Retrieving more data than necessary.
– Solution:
– Use WHERE clause to filter rows based on specific conditions.
sql
-- Filtering data with WHERE clause
SELECT product_name, price
FROM products
WHERE category = 'Electronics';
4. Aggregate Functions and GROUP BY:
– Issue: Inefficient use of aggregate functions.
– Solution:
– Use aggregate functions like COUNT, SUM, AVG judiciously.
– Combine with GROUP BY for better performance.
sql
-- Using COUNT with GROUP BY
SELECT category, COUNT() as product_count
FROM products
GROUP BY category;
5. Subqueries Optimization:
– Issue: Inefficient use of subqueries.
– Solution:
– Replace subqueries with JOINs where applicable.
– Ensure subqueries return a limited result set.
sql
-- Using subquery
SELECT product_name
FROM products
WHERE category_id IN (SELECT category_id FROM categories WHERE category_name = 'Electronics');
6. Avoid SELECT :
– Issue: Retrieving all columns when not necessary.
– Solution:
– Explicitly list only the required columns.
sql
-- Avoiding SELECT
SELECT user_id, username, email
FROM users
WHERE user_type = 'Admin';
7. Query Caching:
– Issue: Redundant execution of identical queries.
– Solution:
– Implement query caching to store results of frequently executed queries.
sql
-- Example of query caching (database-specific implementation)
SELECT SQL_CACHE FROM products WHERE category = 'Clothing';
8. Regular Database Maintenance:
– Issue: Fragmented indexes and outdated statistics.
– Solution:
– Schedule regular database maintenance tasks.
– Update statistics and rebuild indexes.
sql
-- Rebuilding an index
ALTER INDEX idx_username ON users REBUILD;
Conclusion
Optimizing SQL queries is an ongoing process that requires a combination of careful analysis, efficient indexing, and query structure refinement. By implementing the strategies outlined above and continuously monitoring and refining your queries, you’ll ensure that your database-driven applications perform at their best, delivering a seamless experience to users. Remember, each database system may have specific optimization techniques, so tailor your approach based on the database engine you are using.