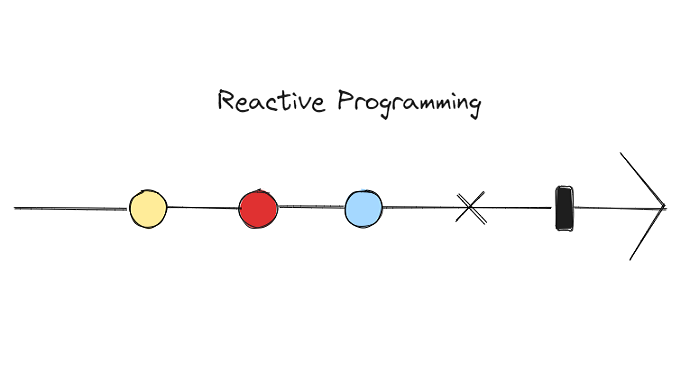
Introduction to Reactive Programming: Concepts and Applications
Reactive Programming is a paradigm that focuses on building applications that can efficiently respond to changes in data and events. It provides a declarative approach to handling asynchronous data streams, making it a powerful tool for creating responsive and scalable systems. In this comprehensive guide, we’ll delve into the core concepts of reactive programming and explore its applications.
Understanding Reactive Programming:
1. Key Concepts:
– Data Streams: In reactive programming, everything is considered as a stream of data, whether it’s user inputs, system events, or asynchronous responses.
– Observer Pattern: The observer pattern is central to reactive programming, where observers (subscribers) react to changes emitted by the source (observable).
2. Reactive Manifesto:
– Responsivene: Systems should respond promptly to user inputs and changes in the environment.
– Resilience: Systems should stay responsive in the face of failures and varying workloads.
– Elasticity: Systems should remain responsive under different load conditions.
Reactive Programming in Action:
3. Frameworks and Libraries:
– RxJava: A popular reactive programming library for Java, providing a rich set of operators to manipulate and combine data streams.
– ReactiveX: A cross-platform library that extends reactive programming concepts to multiple languages, including JavaScript, Python, and more.
4. Benefits of Reactive Programming:
– Asynchronous Operations: Easily manage asynchronous tasks without callbacks, making code more readable and maintainable.
– Data Flow: Create a clear data flow within the application, making it easier to understand and reason about.
Reactive Applications:
5. User Interfaces (UI):
– Responsive UIs: Reactive programming is widely used in front-end development to create responsive user interfaces that react to user inputs in real-time.
– Event Handling: Effortlessly handle complex event-driven scenarios in UI development.
6. Backend Development:
– Scalability: Reactive systems can efficiently handle a large number of concurrent connections, improving scalability.
– Real-time Applications: Implement real-time features in applications, such as chat, notifications, and live updates.
7. IoT (Internet of Things):
– Event Processing: Reactive programming is ideal for processing and reacting to events in IoT devices, providing a flexible and scalable approach.
– Sensor Networks: Manage and react to data streams from sensors in real-time.
Best Practices:
8. Immutable State:
– Avoid Shared State: Minimize shared mutable state to prevent unintended consequences.
– Immutability: Encourage the use of immutable data structures for predictable and reliable applications.
9. Backpressure Handling:
– Buffering: Implement buffering or dropping strategies to handle scenarios where the data producer is faster than the consumer.
– Flow Control: Use backpressure strategies to control the flow of data and prevent overload.
Challenges and Considerations:
10. Learning Curve:
– Paradigm Shift: Reactive programming involves a paradigm shift, and developers may need time to adjust to its concepts.
– Debugging: Debugging asynchronous code can be challenging, and tools and techniques must be adapted.
Future Trends:
11. Integration with Cloud and Microservices:
– Cloud-Native Applications: Integration of reactive programming with cloud-native architectures and microservices for enhanced scalability and responsiveness.
Conclusion:
Reactive programming is a powerful paradigm that provides a systematic way to handle the complexities of asynchronous and event-driven applications. Whether you are developing user interfaces, backend services, or IoT applications, understanding and applying reactive programming concepts can significantly improve the responsiveness and scalability of your systems.
As the technology landscape continues to evolve, reactive programming remains a valuable tool for creating robust and efficient applications.