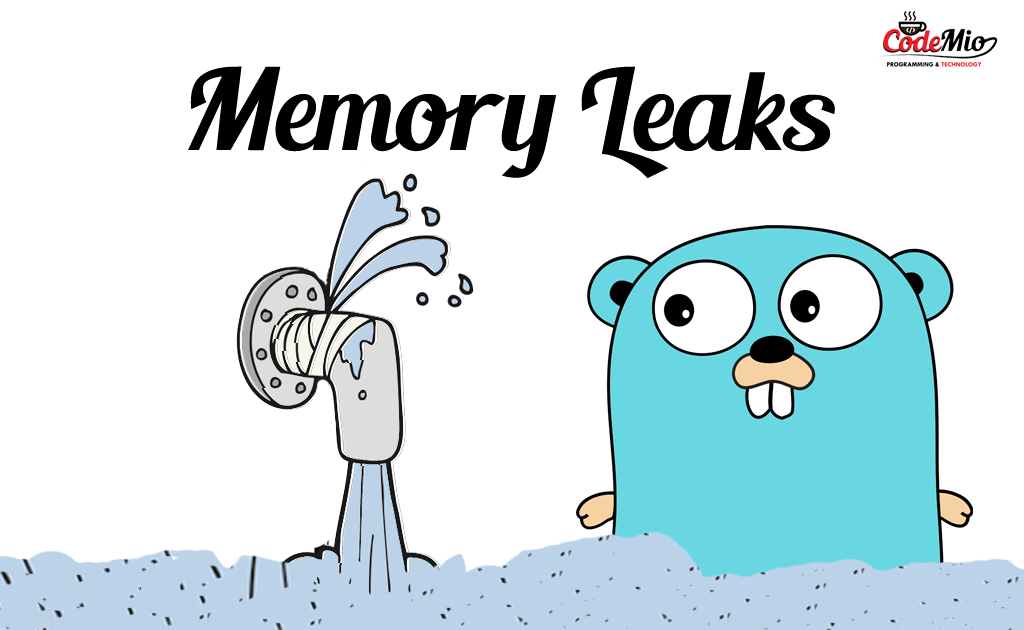
Efficient Memory Management: Tips For Avoiding Memory Leaks In Code
In the intricate realm of software development, efficient memory management is paramount to ensure the optimal performance and stability of applications. Memory leaks, a common challenge, can lead to resource exhaustion and degrade the user experience. This guide delves into the significance of memory management, the perils of memory leaks, and provides practical tips to sidestep these pitfalls.
1. The Significance of Memory Management:
Memory management is the process of controlling and organizing a computer’s memory to facilitate efficient usage by programs. In programming languages like C and C++, developers have explicit control over memory allocation and deallocation, while in higher-level languages like Java or Python, memory management is typically handled by a runtime environment.
Proper memory management offers several advantages:
- Optimal Resource Utilization: Efficient memory usage ensures that the application utilizes the available resources judiciously, preventing unnecessary consumption.
- Prevention of Memory Leaks: Rigorous memory management practices help in identifying and preventing memory leaks, ensuring the application’s stability.
- Enhanced Performance: Well-managed memory contributes to improved application performance, reducing latency and enhancing responsiveness.
2. Understanding Memory Leaks:
A memory leak occurs when a program allocates memory but fails to release it properly, leading to a gradual depletion of available memory. Over time, memory leaks can result in performance degradation, increased resource consumption, and, in extreme cases, application crashes. Common causes of memory leaks include:
- Uncleared Allocated Memory: Failure to deallocate memory after its usage contributes to memory leaks. This often happens when developers forget to release dynamically allocated memory.
- Circular References: In languages with automatic garbage collection, circular references (objects referencing each other) can prevent the garbage collector from reclaiming memory.
- Unreleased Resources: In addition to memory, other resources like file handles or network connections should be released properly to prevent resource leaks.
3. Tips for Avoiding Memory Leaks:
A. Use Automatic Memory Management:
Garbage Collection: In languages with garbage collection (e.g., Java, C#), leverage automatic memory management to let the runtime handle memory cleanup.
B. Explicit Deallocation (C, C++):
Adopt RAII (Resource Acquisition Is Initialization): In C++ and similar languages, use RAII principles to ensure that resources are released when their corresponding objects go out of scope.
Free Allocated Memory: In manual memory management languages (C, C++), diligently free dynamically allocated memory when it is no longer needed.
C. Tools for Memory Analysis:
Memory Profilers: Employ memory profiling tools to analyze memory usage patterns, identify leaks, and optimize memory-heavy sections of code.
Static Code Analysis: Use static code analysis tools to catch potential memory issues during the development phase.
D. Regular Code Reviews:
Peer Reviews: Conduct regular code reviews, with a focus on memory management practices. Fresh perspectives can help spot potential issues.
Check for Resource Cleanup: Specifically check for proper release of resources, including memory, file handles, and other critical resources.
E. Leverage Smart Pointers:
Use Smart Pointers (C++): In C++, prefer smart pointers like std::shared_ptr or std::unique_ptr to manage memory automatically, reducing the risk of leaks.
F. Monitor Resource Utilization:
Resource Monitoring Tools:Implement resource monitoring tools to track memory usage and identify abnormal patterns indicative of potential leaks.
G. Handle Circular References:
Weak References: When dealing with circular references, use weak references to break the cycle, allowing the garbage collector to reclaim memory.
H. Comprehensive Testing:
Memory Tests: Include memory tests as part of your testing strategy to catch memory leaks through automated testing.
I. Continuous Learning:
Stay Informed: Keep abreast of best practices and new techniques for memory management. Continuous learning ensures that your approach aligns with industry standards.
4. Conclusion:
Efficient memory management is foundational to robust software development. By understanding the importance of proper memory handling and adopting the tips outlined in this guide, developers can significantly mitigate the risk of memory leaks. Vigilance, regular code reviews, and the use of appropriate tools contribute to the creation of applications that not only perform optimally but also provide a seamless and reliable user experience.
Efficient memory management is not just a technical consideration; it’s a cornerstone of delivering high-quality software solutions.