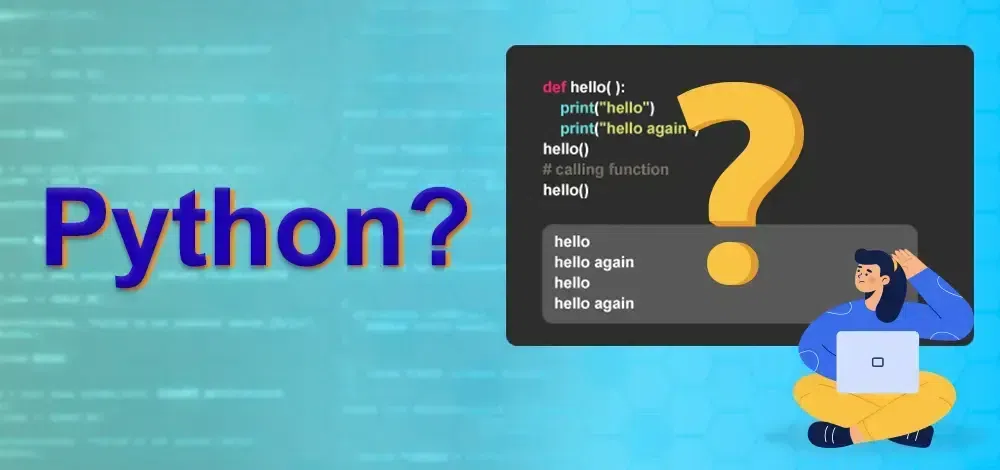
Deep Dive into Python: Tips and Tricks for Developers
Python, known for its simplicity and versatility, has become a powerhouse in the programming world. Whether you’re a seasoned Python developer or just starting your journey, diving deep into Python can unearth a wealth of tips and tricks that enhance your coding experience. In this comprehensive guide, we’ll explore advanced techniques, best practices, and lesser-known features to elevate your Python skills.
1. Mastering List Comprehensions:
– Description: Take advantage of concise and powerful list comprehensions for creating lists in a single line.
– Example:
python
squares = [x2 for x in range(10)]
2. Understanding Generators and Iterators:
– Description: Learn to use generators for lazy evaluation, saving memory by producing values on-the-fly.
– Example:
python
def square_generator(n):
for i in range(n):
yield i2
3. Context Managers with `with` Statement:
– Description: Leverage the `with` statement and context managers for resource management.
– Example:
python
with open('file.txt', 'r') as file:
content = file.read()
4. Decorators for Code Reusability:
– Description: Use decorators to modify or extend the behavior of functions, promoting code reusability.
– Example:
python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
5. Working with `collections` Module:
– Description: Explore the `collections` module for specialized data structures beyond the built-in types.
– Example:
python
from collections import Counter
word_count = Counter(['apple', 'banana', 'apple', 'orange'])
6. Utilizing `functools` for Higher-Order Functions:
– Description: Employ `functools` for working with higher-order functions and function manipulation.
– Example:
python
from functools import partial
multiply = partial(lambda x, y: x y, 2)
result = multiply(5) Result: 10
7. Concurrency with `asyncio`:
– Description: Embrace asynchronous programming using the `asyncio` module for efficient I/O-bound operations.
– Example:
python
import asyncio
async def my_async_function():
Asynchronous operations here
asyncio.run(my_async_function())
8. Exploring `itertools` for Iterative Operations:
– Description: Dive into the `itertools` module for iterators and functions to work with them efficiently.
– Example:
python
from itertools import combinations
combinations_result = combinations([1, 2, 3], 2)
9. Working with `__dunder__` Methods:
– Description: Understand and leverage special methods (dunder methods) to customize class behavior.
– Example:
python
class MyClass:
def __init__(self, value):
self.value = value
def __repr__(self):
return f"MyClass({self.value})"
10. Using `Enum` for Readable Constants:
– Description: Enhance code readability by using enumerations to represent named, constant values.
– Example:
python
from enum import Enum
class Color(Enum):
RED = 1
GREEN = 2
BLUE = 3
11. Efficient String Formatting:
– Description: Explore different string formatting techniques for improved readability and performance.
– Example:
python
name = "John"
age = 30
formatted_string = f"Name: {name}, Age: {age}"
12. Working with `zip` for Parallel Iteration:
– Description: Use the `zip` function for parallel iteration over multiple iterables.
– Example:
python
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
for name, age in zip(names, ages):
print(f"Name: {name}, Age: {age}")
13. Optimizing Performance with `cython`:
– Description: Utilize `cython` to boost performance by compiling Python code into C extensions.
– Example:
python
my_module.pyx
def my_function():
Some Python code
setup.py
from setuptools import setup
from Cython.Build import cythonize
setup(ext_modules = cythonize("my_module.pyx"))
14. Interactive Debugging with `pdb`:
– Description: Master the Python Debugger (`pdb`) for interactive debugging and troubleshooting.
– Example:
python
import pdb
def my_function():
pdb.set_trace()
Code execution will pause, allowing interactive inspection
15. Enhancing Documentation with `doc
strings`:
– Description: Write informative and standardized docstrings to improve code documentation.
– Example:
python
def my_function(param1, param2):
"""
Description of the function.
Parameters:
- param1: Explanation of param1.
- param2: Explanation of param2.
Returns:
Explanation of the return value.
"""
Function implementation
Conclusion: Unlocking the Full Potential of Python
Python’s richness lies not just in its simplicity but in the myriad features and capabilities it offers. By delving into these advanced tips and tricks, developers can unlock the full potential of Python and write more efficient, readable, and maintainable code. Whether you’re building web applications, data science projects, or automation scripts, mastering these techniques will elevate your Python programming skills and make you a more proficient developer.
Happy coding!