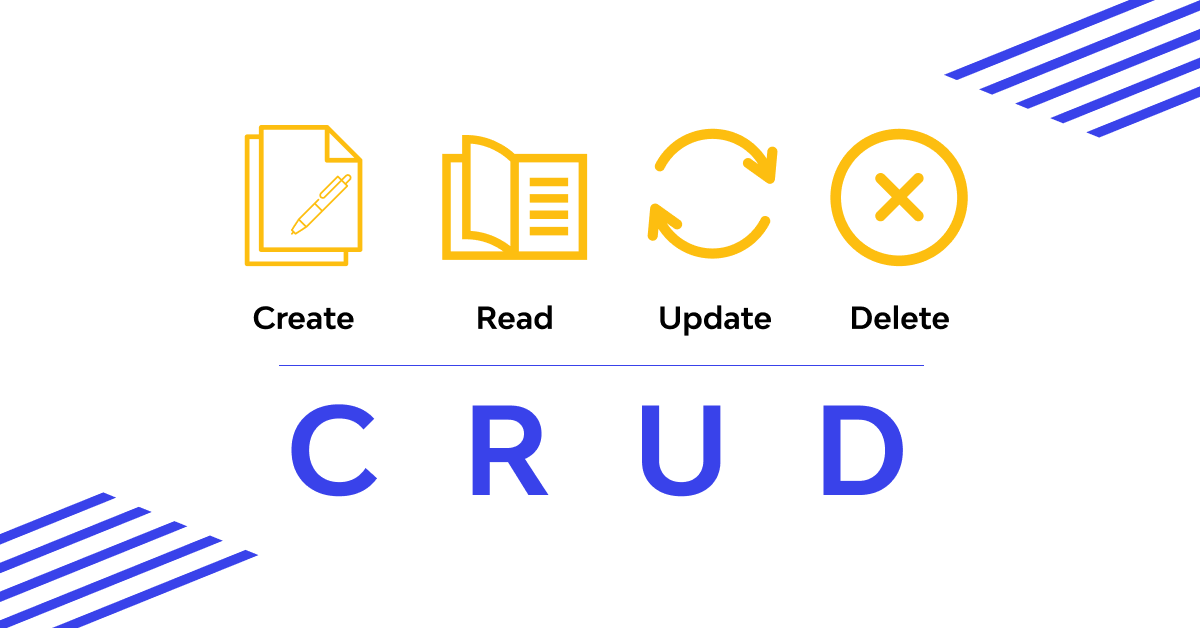
Creating a Simple CRUD Application in React: Code Walkthrough
Building a CRUD (Create, Read, Update, Delete) application is a fundamental exercise in web development, providing hands-on experience with front-end and back-end interactions. In this guide, we’ll focus on creating a simple CRUD application using the React framework. We’ll walk through each step of the process, from setting up the project to implementing the core functionality.
Prerequisites
Before we begin, ensure that you have Node.js and npm (Node Package Manager) installed on your machine. Additionally, you may need a code editor; Visual Studio Code is recommended.
Step 1: Set Up the React Project
Open your terminal and run the following commands:
bash
npx create-react-app react-crud-app
cd react-crud-app
This will create a new React project and navigate to its directory.
Step 2: Install Dependencies
Install additional dependencies for state management and routing:
bash
npm install axios react-router-dom
Axios will be used for making HTTP requests, and React Router will handle navigation.
Step 3: Project Structure
Create a basic project structure:
plaintext
src
|-- components
| |-- Create.js
| |-- Read.js
| |-- Update.js
| |-- Delete.js
|-- App.js
|-- index.js
Step 4: Implement CRUD Functionality
Create.js
import React, { useState } from 'react';
import axios from 'axios';
const Create = () => {
const [data, setData] = useState({});
const handleCreate = async () => {
try {
// Make a POST request to create data
const response = await axios.post('https://api.example.com/data', data);
console.log('Data created:', response.data);
} catch (error) {
console.error('Error creating data:', error);
}
};
return (
<div>
<h2>Create Data</h2>
{/ Form inputs and submit button /}
</div>
);
};
export default Create;
Read.js`
```jsx
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const Read = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
// Make a GET request to fetch data
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
return (
<div>
<h2>Read Data</h2>
{/ Display fetched data /}
</div>
);
};
export default Read;
Update.js
import React, { useState } from 'react';
import axios from 'axios';
const Update = () => {
const [data, setData] = useState({});
const handleUpdate = async () => {
try {
// Make a PUT request to update data
const response = await axios.put('https://api.example.com/data/1', data);
console.log('Data updated:', response.data);
} catch (error) {
console.error('Error updating data:', error);
}
};
return (
<div>
<h2>Update Data</h2>
{/ Form inputs, fetch data to update, and submit button /}
</div>
);
};
export default Update;
Delete.js
import React from 'react';
import axios from 'axios';
const Delete = () => {
const handleDelete = async () => {
try {
// Make a DELETE request to delete data
const response = await axios.delete('https://api.example.com/data/1');
console.log('Data deleted:', response.data);
} catch (error) {
console.error('Error deleting data:', error);
}
};
return (
<div>
<h2>Delete Data</h2>
{/ Confirm deletion and delete button /}
</div>
);
};
export default Delete;
App.js
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
import Create from './components/Create';
import Read from './components/Read';
import Update from './components/Update';
import Delete from './components/Delete';
const App = () => {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/create">Create</Link></li>
<li><Link to="/read">Read</Link></li>
<li><Link to="/update">Update</Link></li>
<li><Link to="/delete">Delete</Link></li>
</ul>
</nav>
<Route path="/create" exact component={Create} />
<Route path="/read" exact component={Read} />
<Route path="/update" exact component={Update} />
<Route path="/delete" exact component={Delete} />
</div>
</Router>
);
};
export default App;
Step 5: Run the Application
In the terminal, run:
bash
npm start
This will start the development server, and you can access the application at [http://localhost:3000].
Congratulations! You’ve successfully created a simple CRUD application using React. This walkthrough provides a foundation that you can build upon by adding more features, enhancing styling, or integrating with a backend server.
Happy coding!