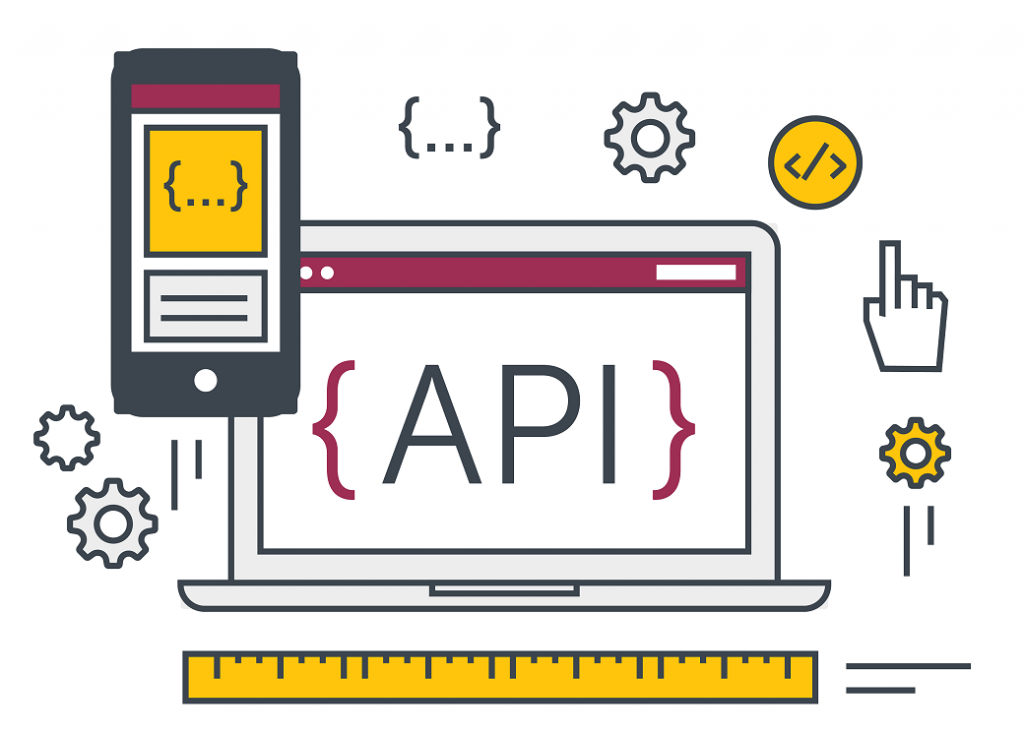
Building a RESTful API from Scratch: Code Walkthrough and Best Practices
Building a RESTful API from scratch is a rewarding endeavor that empowers developers to create scalable and efficient web services. In this guide, we’ll walk through the process step-by-step, covering essential concepts and best practices along the way.
Step 1: Define Your API
Before writing code, clearly define the purpose and functionality of your API. Identify the resources it will expose and the actions that can be performed on those resources. For example, if building a blog API, resources could include `posts` and `comments`.
Step 2: Set Up Your Development Environment
Ensure you have a working development environment with a suitable code editor, version control system (e.g., Git), and the programming language of your choice. For this walkthrough, we’ll use Node.js with Express.
Step 3: Initialize Your Project
Create a new directory for your project and initialize a new Node.js project with:
bash
: npm init -y
Install Express:
bash: npm install express
Step 4: Create Your Express App
Set up a basic Express app in your `index.js` file:
javascript
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
// Define routes here
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
Step 5: Define API Routes
Create routes for your resources. For example, for a blog API:
javascript
const posts = [
{ id: 1, title: 'First Post', content: 'This is the content of the first post.' },
// Add more posts as needed
];
// Get all posts
app.get('/posts', (req, res) => {
res.json(posts);
});
// Get a specific post
app.get('/posts/:id', (req, res) => {
const post = posts.find(p => p.id === parseInt(req.params.id));
if (!post) return res.status(404).json({ message: 'Post not found' });
res.json(post);
});
// Create a new post
app.post('/posts', (req, res) => {
const newPost = req.body;
posts.push(newPost);
res.status(201).json(newPost);
});
// Add routes for updating and deleting posts as needed
Step 6: Implement RESTful Practices
Follow RESTful principles:
– Use appropriate HTTP methods (GET, POST, PUT, DELETE).
– Use plural nouns for resource names (`/posts` instead of `/post`).
– Use HTTP status codes correctly (e.g., 200 OK, 201 Created, 404 Not Found).
Step 7: Test Your API
Use tools like Postman or curl to test your API routes. Ensure that endpoints return the expected results and handle errors appropriately.
Step 8: Implement Validation and Error Handling
Add input validation and proper error handling to enhance the robustness of your API.
Step 9: Document Your API
Create clear and concise documentation that explains how to use your API. Tools like Swagger or API Blueprint can assist in generating API documentation.
Step 10: Secure Your API
Implement security best practices, including input validation, authentication, and authorization. Consider using HTTPS to encrypt data transmitted between clients and your API.
Conclusion
Building a RESTful API involves careful planning, adherence to best practices, and continuous testing and refinement. By following this walkthrough and incorporating these best practices, you’ll be well on your way to creating a robust and efficient API for your applications. Happy coding!