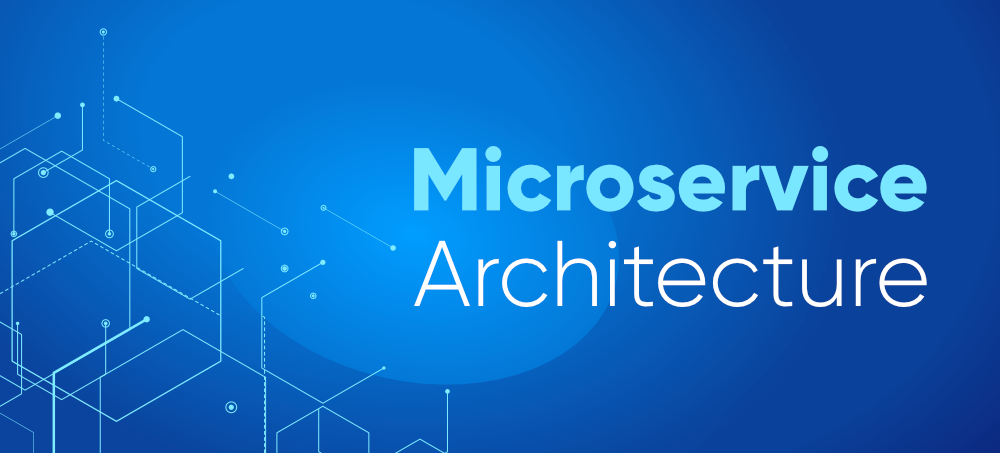
Best Practices for Building Scalable Microservices Architecture
In the era of modern software development, microservices architecture has gained prominence for its ability to create flexible, scalable, and maintainable systems. However, harnessing the full potential of microservices requires adherence to best practices that ensure robustness, scalability, and ease of management. In this guide, we explore key principles and practices for building a scalable microservices architecture.
1. Decompose Monoliths Thoughtfully:
– Modular Design: Break down monolithic applications into independent, loosely coupled modules that can evolve independently.
– Identify Bounded Contexts: Define clear boundaries between microservices by identifying bounded contexts to avoid unnecessary dependencies.
2. Design Autonomous Services:
– Isolation of Concerns: Ensure that each microservice has a well-defined purpose and handles a specific business capability.
– Independent Data Management: Microservices should manage their data storage independently, avoiding shared databases to prevent tight coupling.
3. API First and Contract-First Development:
– API Contracts: Design APIs before implementation, fostering a contract-first approach to ensure interoperability and consistency.
– Versioning: Implement API versioning to manage changes without affecting existing consumers.
4. Resilience and Fault Tolerance:
– Circuit Breaker Pattern: Implement circuit breakers to prevent cascading failures and provide graceful degradation during service outages.
– Retry Strategies: Configure intelligent retry mechanisms to handle transient failures and maintain system availability.
5. Scalability and Load Balancing:
– Horizontal Scaling: Design microservices to scale horizontally, allowing the addition of instances to handle increased load.
– Load Balancers: Utilize load balancers to distribute incoming traffic evenly across microservices instances.
6. Containerization and Orchestration:
– Docker Containers: Containerize microservices using technologies like Docker to ensure consistency across development, testing, and production.
– Kubernetes Orchestration: Leverage Kubernetes for container orchestration, automating deployment, scaling, and management of microservices.
7. Centralized Configuration Management:
– Externalized Configuration: Externalize configuration settings, allowing microservices to adapt to different environments without code changes.
– Configuration Servers: Implement centralized configuration servers to manage configuration data dynamically.
8. Monitoring and Observability:
– Distributed Tracing: Use distributed tracing tools to monitor and trace requests as they traverse through microservices.
– Centralized Logging: Aggregate logs centrally for easier troubleshooting and performance analysis.
9. Security Measures:
– Token-Based Authentication: Implement token-based authentication for securing communication between microservices.
– Role-Based Access Control (RBAC): Enforce RBAC to manage permissions and access control effectively.
10. Continuous Integration and Deployment (CI/CD):
– Automated Pipelines:
– Set up CI/CD pipelines to automate testing, building, and deploying microservices consistently.
– Blue-Green Deployments:
– Utilize blue-green deployments to minimize downtime during updates and releases.
11. Documentation and Communication:
– API Documentation: Maintain comprehensive and up-to-date documentation for each microservice API.
– Inter-Service Communication: Clearly define protocols and communication patterns between microservices.
12. Testing Strategies:
– Unit Testing:
– Implement robust unit testing for each microservice to ensure individual components function correctly.
– Contract Testing: Conduct contract testing to validate interactions between microservices.
13. Evolutionary Architecture:
– Graceful Deprecation:
– Plan for the evolution of microservices by deprecating features gracefully.
– Implement fallback mechanisms for backward compatibility.
14. Team Autonomy:
– Cross-Functional Teams: Form cross-functional teams, each responsible for a set of microservices, promoting autonomy and accountability.
– DevOps Culture: Cultivate a DevOps culture to bridge the gap between development and operations for seamless collaboration.
15. Performance Optimization:
– Database Indexing: Optimize database performance with proper indexing and query optimization.
– Caching Strategies: Implement caching mechanisms to reduce latency and enhance overall system performance.
Building a scalable microservices architecture requires a holistic approach, integrating principles of design, resilience, security, and automation. By adhering to these best practices, development teams can navigate the complexities of microservices and unlock the potential for agility, scalability, and robustness in their systems.